An AI Code Assistant for Rapid Development
on
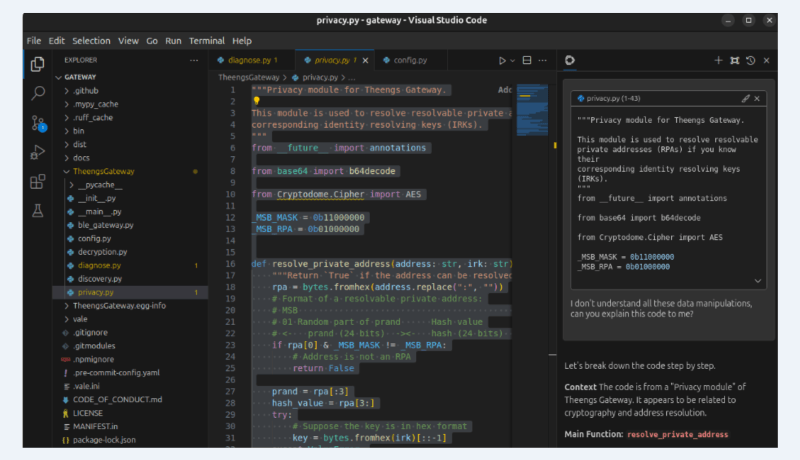
Accelerate software development with large language models! This tutorial explores how to leverage the Continue extension for Visual Studio Code, showcasing the benefits of an AI code assistant that excels at autocompleting, rewriting, and explaining code.
Large language models (LLM) like OpenAI’s ChatGPT are often referred to as “autocomplete on steroids” for a variety of programming languages, but they offer much more. While these neural networks essentially predict and complete the text you type, training them on extensive data sets of texts and conversations has resulted in unexpected emergent capabilities. You can even run the LLM entirely on your own hardware, ensuring that you don’t send confidential information to the cloud.
One remarkable capability is their proficiency in coding tasks. You can instruct an LLM to draft a shell script for a one-off task, refactor existing code, shed light on a compilation error, or even generate unit tests. LLMs are especially useful w...
Continue reading this Elektor article?
- Try a digital membership (Green) for 4 months for only 1.75 per month.
- Limited access to Elektor archive, digital magazines and over 5,000 Gerber files.
- Independent reviews and high-quality DIY electronics projects. Inspiration and solutions for all your electronics challenges.
- A community of active e-engineers – from beginners to professionals.
